If you’re reading this article, it might have happened to you as well that you wanted to check if a list of URLs is indexed on Google, but Google Search Console allows you to process one at a time only.
Is there a way to paste or import a list of URLs and check them all together?
Unfortunately, not officially. Google Search Console does not allow you to verify URLs in bulk.
However, you can get past this roadblock, inject a script inside the URL inspection page, and do it anyway.
Want to know how?
How to check if the list of URLs is indexed on Google?
Using the browser console to paste a script into the Google Search Console website is a lightweight way to automate URL indexing checks. This method is limited compared to leveraging a library like Puppeteer, but it works well for small-scale automation.
Here’s how you can do it.
Check if URLs are indexed on Google Search Console (step-by-step guide)
1. Prepare your URL list
- Create a list of URLs you want to check. You can store them in a JavaScript array directly in the script (more on this later).
2. Open Google Search Console
- Go to the URL Inspection Tool in Google Search Console: https://search.google.com/search-console/url-inspection.
- Ensure you are logged into your Google account.
3. Inspect the URL input field
- Right-click on the URL search bar in Google Search Console, then click on Inspect in the browser’s dropdown menu that appears.
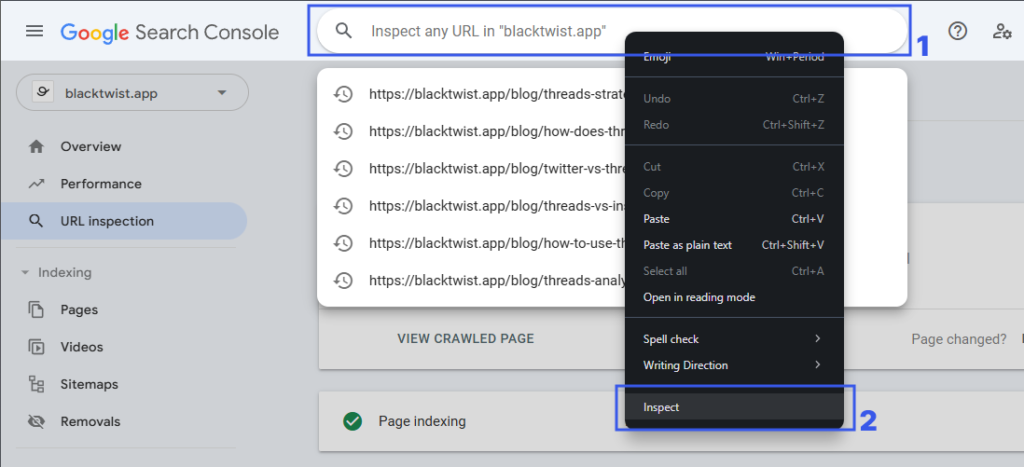
- Once done, take note of the input class. In this example, it’s “
Ax4B8 ZAGvjd
” but consider that it changes every time (Google scrambles, or better, obfuscates their CSS classes).
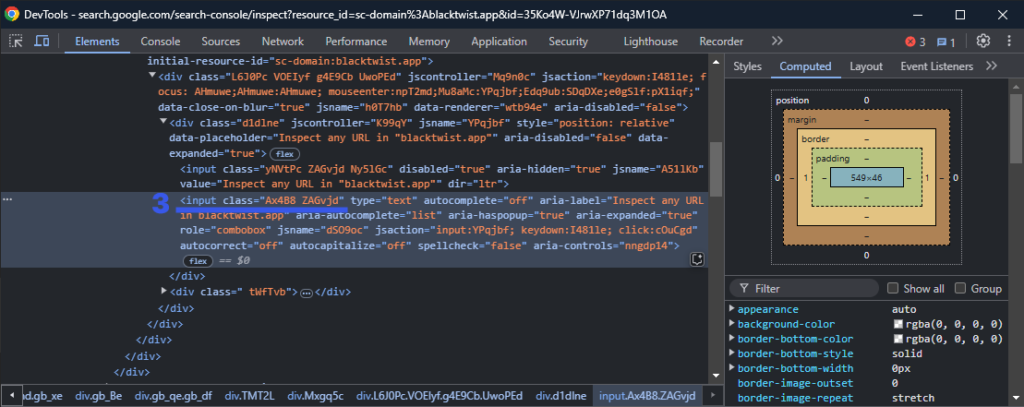
4. Open the Developer Tools
- Press
F12
(orCtrl+Shift+I
/Cmd+Opt+I
on Mac) to open the Developer Tools. - Navigate to the Console tab.
- Don’t worry if you see a warning message—what we are doing here is totally safe.
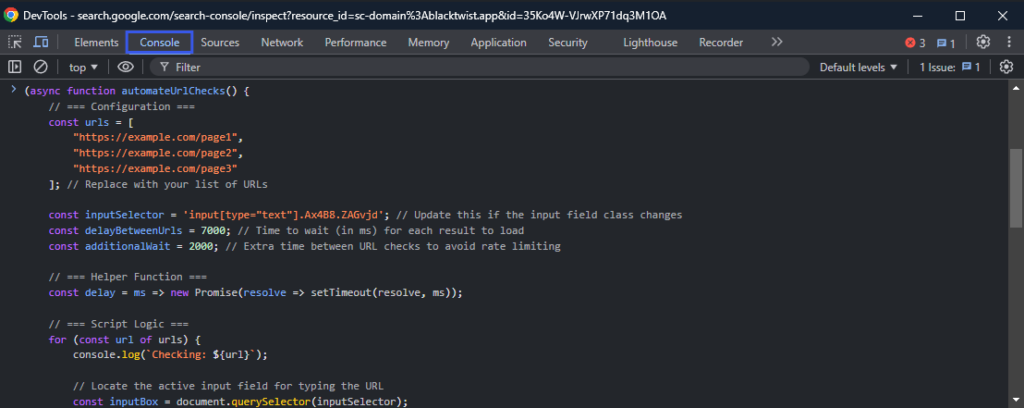
5. Paste and run the script
- Copy the script below.
- Make sure to switch the list of example URLs with the URLs you want to check.
- Change the
inputSelector
class with the one you’ve noted before (in my case, it was “Ax4B8 ZAGvjd
” and it became “input[type="text"].Ax4B8.ZAGvjd
” as you can see below). Make sure to add yours instead.
(async function automateUrlChecks() {
// === Configuration ===
const urls = [
"https://example.com/page1",
"https://example.com/page2",
"https://example.com/page3"
]; // Replace with your list of URLs
const inputSelector = 'input[type="text"].Ax4B8.ZAGvjd'; // Update this if the input field class changes
const delayBetweenUrls = 7000; // Time to wait (in ms) for each result to load
const additionalWait = 2000; // Extra time between URL checks to avoid rate limiting
// === Helper Function ===
const delay = ms => new Promise(resolve => setTimeout(resolve, ms));
// === Script Logic ===
for (const url of urls) {
console.log(`Checking: ${url}`);
// Locate the active input field for typing the URL
const inputBox = document.querySelector(inputSelector);
if (!inputBox) {
console.error("Input box not found! Make sure you're on the URL Inspection page.");
return;
}
// Set the value and trigger an input event
inputBox.value = url;
inputBox.dispatchEvent(new Event('input', { bubbles: true }));
// Wait briefly to ensure input is processed
await delay(1000);
// Simulate pressing Enter
inputBox.dispatchEvent(new KeyboardEvent('keydown', {
key: 'Enter',
code: 'Enter',
keyCode: 13, // For compatibility
bubbles: true,
cancelable: true
}));
console.log("Triggered Enter key...");
// Wait for the results to load
console.log("Waiting for the results...");
await delay(delayBetweenUrls);
// Placeholder: Optionally extract results here
const resultContainer = document.querySelector('.result-container'); // Adjust this if needed
if (resultContainer) {
console.log(`Result for ${url}: ${resultContainer.textContent.trim()}`);
} else {
console.error(`Result for ${url} not found. Check manually on the page.`);
}
// Wait before processing the next URL
console.log("Waiting before the next URL...");
await delay(additionalWait);
}
console.log("URL checks complete!");
})();
- Now, paste it inside your console tab.
- Press
Enter
on your keyboard to run the script. - Watch the magic happen!
Remember to take notes about the URLs that are not indexed so you can get back later and fix them. I’ve done this inside a Google Sheets file.
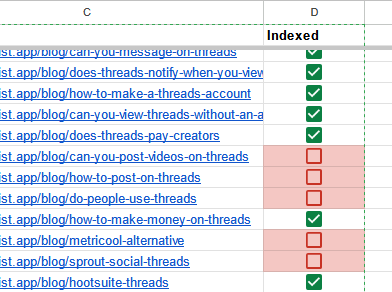
Considerations
- The
resultContainer
extraction logic is marked as a placeholder. You can replace the selector or add your own logic if you want to log results programmatically.
- The
urls
array andinputSelector
variables are clearly defined at the top, making it easy to replace them with your own URLs and input field selector.
- You can easily tweak
delayBetweenUrls
andadditionalWait
for timing adjustments without digging into the script.